mirror of
https://git.mills.io/saltyim/saltyim.git
synced 2024-06-16 03:48:24 +00:00
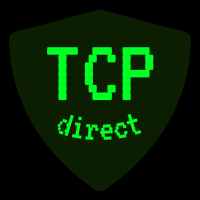
Co-authored-by: Jon Lundy <jon@xuu.cc> Reviewed-on: https://git.mills.io/saltyim/saltyim/pulls/175 Reviewed-by: James Mills <james@mills.io> Co-authored-by: xuu <xuu@noreply@mills.io> Co-committed-by: xuu <xuu@noreply@mills.io>
155 lines
3.7 KiB
Go
155 lines
3.7 KiB
Go
package internal
|
|
|
|
import (
|
|
"fmt"
|
|
"net/url"
|
|
"os"
|
|
)
|
|
|
|
const (
|
|
serviceUser = "salty"
|
|
|
|
// InvalidConfigValue is the constant value for invalid config values
|
|
// which must be changed for production configurations before successful
|
|
// startup
|
|
InvalidConfigValue = "INVALID CONFIG VALUE - PLEASE CHANGE THIS VALUE"
|
|
|
|
// DefaultDebug is the default debug mode
|
|
DefaultDebug = false
|
|
|
|
// DefaultTLS is the default for whether to enable TLS
|
|
DefaultTLS = false
|
|
|
|
// DefaultTLSKey is the default path to a TLS private key (if blank uses Let's Encrypt)
|
|
DefaultTLSKey = ""
|
|
|
|
// DefaultTLSCert is the default path to a TLS certificate (if blank uses Let's Encrypt)
|
|
DefaultTLSCert = ""
|
|
|
|
// DefaultData is the default data directory for storage
|
|
DefaultData = "./data"
|
|
|
|
// DefaultStore is the default data store used for accounts, sessions, etc
|
|
DefaultStore = "bitcask://data/saltyim.db"
|
|
|
|
// DefaultBaseURL is the default Base URL for the server
|
|
DefaultBaseURL = "https://salty." + DefaultPrimaryDomain
|
|
|
|
// DefaultPrimaryDomain is the default primary domain delegated to this broker
|
|
DefaultPrimaryDomain = "home.arpa"
|
|
|
|
// DefaultAdminUser is the default publickye to grant admin privileges to
|
|
DefaultAdminUser = ""
|
|
|
|
// DefaultSupportEmail is the default email of the admin user used in support requests
|
|
DefaultSupportEmail = "support@" + DefaultPrimaryDomain
|
|
)
|
|
|
|
func NewConfig() *Config {
|
|
return &Config{
|
|
Debug: DefaultDebug,
|
|
Store: DefaultStore,
|
|
|
|
BaseURL: DefaultBaseURL,
|
|
PrimaryDomain: DefaultPrimaryDomain,
|
|
|
|
AdminUser: DefaultAdminUser,
|
|
SupportEmail: DefaultSupportEmail,
|
|
}
|
|
}
|
|
|
|
// Option is a function that takes a config struct and modifies it
|
|
type Option func(*Config) error
|
|
|
|
// WithDebug sets the debug mode lfag
|
|
func WithDebug(debug bool) Option {
|
|
return func(cfg *Config) error {
|
|
cfg.Debug = debug
|
|
return nil
|
|
}
|
|
}
|
|
|
|
// WithTLS sets the tls flag
|
|
func WithTLS(tls bool) Option {
|
|
return func(cfg *Config) error {
|
|
cfg.TLS = tls
|
|
return nil
|
|
}
|
|
}
|
|
|
|
// WithTLSKey sets the path to a TLS private key
|
|
func WithTLSKey(tlsKey string) Option {
|
|
return func(cfg *Config) error {
|
|
cfg.TLSKey = tlsKey
|
|
return nil
|
|
}
|
|
}
|
|
|
|
// WithTLSCert sets the path to a TLS certificate
|
|
func WithTLSCert(tlsCert string) Option {
|
|
return func(cfg *Config) error {
|
|
cfg.TLSCert = tlsCert
|
|
return nil
|
|
}
|
|
}
|
|
|
|
// WithData sets the data directory to use for storage
|
|
func WithData(data string) Option {
|
|
return func(cfg *Config) error {
|
|
if err := os.MkdirAll(data, 0755); err != nil {
|
|
return fmt.Errorf("error creating data directory %s: %w", data, err)
|
|
}
|
|
cfg.Data = data
|
|
return nil
|
|
}
|
|
}
|
|
|
|
// WithStore sets the store to use for accounts, sessions, etc.
|
|
func WithStore(store string) Option {
|
|
return func(cfg *Config) error {
|
|
cfg.Store = store
|
|
return nil
|
|
}
|
|
}
|
|
|
|
// WithBaseURL sets the Base URL used for constructing feed URLs
|
|
func WithBaseURL(baseURL string) Option {
|
|
return func(cfg *Config) error {
|
|
u, err := url.Parse(baseURL)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
cfg.BaseURL = baseURL
|
|
if baseURL != DefaultBaseURL {
|
|
cfg.BrokerURI = baseURL
|
|
}
|
|
|
|
cfg.baseURL = u
|
|
return nil
|
|
}
|
|
}
|
|
|
|
// WithPrimaryDomain sets the Primary Domain this broker is delegated for
|
|
func WithPrimaryDomain(primaryDomain string) Option {
|
|
return func(cfg *Config) error {
|
|
cfg.PrimaryDomain = primaryDomain
|
|
return nil
|
|
}
|
|
}
|
|
|
|
// WithAdminUser sets the Admin user used for granting special features to
|
|
func WithAdminUser(adminUser string) Option {
|
|
return func(cfg *Config) error {
|
|
cfg.AdminUser = adminUser
|
|
return nil
|
|
}
|
|
}
|
|
|
|
// WithSupportEmail sets the Support email used to contact the operator of this broker
|
|
func WithSupportEmail(supportEmail string) Option {
|
|
return func(cfg *Config) error {
|
|
cfg.SupportEmail = supportEmail
|
|
return nil
|
|
}
|
|
}
|