mirror of
https://git.mills.io/saltyim/saltyim.git
synced 2024-06-30 18:51:03 +00:00
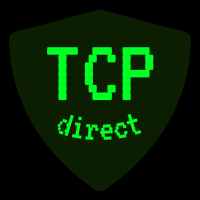
 trying out message formatting in the style of text message chatting where incoming is left justified and send messages are right justified only the last message will have a timestamp WIP until onClick displays timestamp of message Co-authored-by: mlctrez <mlctrez@gmail.com> Reviewed-on: https://git.mills.io/saltyim/saltyim/pulls/102 Reviewed-by: xuu <xuu@noreply@mills.io> Co-authored-by: mlctrez <mlctrez@noreply@mills.io> Co-committed-by: mlctrez <mlctrez@noreply@mills.io>
83 lines
1.9 KiB
Go
83 lines
1.9 KiB
Go
package components
|
|
|
|
import (
|
|
"fmt"
|
|
|
|
"github.com/maxence-charriere/go-app/v9/pkg/app"
|
|
"github.com/mlctrez/goapp-mdc/pkg/base"
|
|
"go.mills.io/saltyim/internal/pwa/storage"
|
|
"go.yarn.social/lextwt"
|
|
)
|
|
|
|
type ChatBox struct {
|
|
app.Compo
|
|
base.JsUtil
|
|
// User is who we're conversing with
|
|
User string
|
|
messages []string
|
|
}
|
|
|
|
func (c *ChatBox) OnMount(ctx app.Context) {
|
|
ctx.Handle("chatbox", c.actionHandler)
|
|
if c.User != "" {
|
|
c.messages = storage.ConversationsLocalStorage(ctx, c.User).Read()
|
|
}
|
|
ctx.Defer(func(context app.Context) {
|
|
c.Update()
|
|
c.scrollChatPane(ctx)
|
|
})
|
|
}
|
|
|
|
// actionHandler receives messages intended to update this component
|
|
func (c *ChatBox) actionHandler(ctx app.Context, action app.Action) {
|
|
c.User = action.Tags.Get("user")
|
|
c.messages = storage.ConversationsLocalStorage(ctx, c.User).Read()
|
|
c.Update()
|
|
}
|
|
|
|
func (c *ChatBox) UpdateMessages(ctx app.Context) {
|
|
if c.User == "" {
|
|
return
|
|
}
|
|
ctx.NewAction("chatbox", app.T("user", c.User))
|
|
c.scrollChatPane(ctx)
|
|
}
|
|
|
|
func (c *ChatBox) Render() app.UI {
|
|
return app.Div().ID("chatbox").Body(c.body())
|
|
}
|
|
|
|
func (c *ChatBox) body() app.UI {
|
|
if c.User == "" {
|
|
return app.P().Text("no user selected to chat with")
|
|
}
|
|
if len(c.messages) == 0 {
|
|
return app.P().Text(fmt.Sprintf("no messages for user = %q", c.User))
|
|
} else {
|
|
return app.Range(c.messages).Slice(func(i int) app.UI {
|
|
s, err := lextwt.ParseSalty(c.messages[i])
|
|
if err != nil {
|
|
app.P().Class("chat-paragraph").Text(c.messages[i])
|
|
}
|
|
switch sc := s.(type) {
|
|
case *lextwt.SaltyText:
|
|
self := !(sc.User.String() == c.User)
|
|
return &ChatMessage{Self: self, Message: sc}
|
|
default:
|
|
return nil
|
|
}
|
|
})
|
|
}
|
|
}
|
|
|
|
func (c *ChatBox) OnResize(ctx app.Context) {
|
|
c.scrollChatPane(ctx)
|
|
}
|
|
|
|
func (c *ChatBox) scrollChatPane(ctx app.Context) {
|
|
ctx.Defer(func(context app.Context) {
|
|
chatBoxDiv := c.JsUtil.JsValueAtPath("chatbox")
|
|
chatBoxDiv.Set("scrollTop", chatBoxDiv.Get("scrollHeight"))
|
|
})
|
|
}
|