mirror of
https://git.mills.io/saltyim/saltyim.git
synced 2024-06-16 11:58:24 +00:00
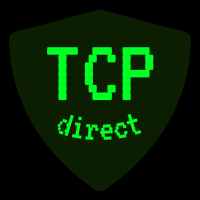
Co-authored-by: James Mills <prologic@shortcircuit.net.au> Reviewed-on: https://git.mills.io/saltyim/saltyim/pulls/147 Reviewed-by: xuu <xuu@noreply@mills.io> Co-authored-by: James Mills <james@mills.io> Co-committed-by: James Mills <james@mills.io>
80 lines
2.0 KiB
Go
80 lines
2.0 KiB
Go
package saltyim
|
|
|
|
import (
|
|
"encoding/json"
|
|
"io"
|
|
"io/ioutil"
|
|
|
|
"go.mills.io/salty"
|
|
)
|
|
|
|
// RegisterRequest is the request used by clients to register to a broker
|
|
type RegisterRequest struct {
|
|
Hash string
|
|
Key string
|
|
}
|
|
|
|
// NewRegisterRequest reads the signed request body from a client, verifies its signature
|
|
// and returns the resulting `RegisterRequest` and key used to sign the request on success
|
|
// otherwise an empty object and en error on failure.
|
|
func NewRegisterRequest(r io.Reader) (req RegisterRequest, signer string, err error) {
|
|
body, err := ioutil.ReadAll(r)
|
|
if err != nil {
|
|
return
|
|
}
|
|
out, key, err := salty.Verify(body)
|
|
if err != nil {
|
|
return
|
|
}
|
|
signer = key.ID().String()
|
|
err = json.Unmarshal(out, &req)
|
|
return
|
|
}
|
|
|
|
// SendRequest is the request used by clients to send messages via a broker
|
|
type SendRequest struct {
|
|
Endpoint string
|
|
Message string
|
|
Capabilities Capabilities
|
|
}
|
|
|
|
// NewSendRequest reads the signed request body from a client, verifies its signature
|
|
// and returns the resulting `SendRequest` and key used to sign the request on success
|
|
// otherwise an empty object and en error on failure.
|
|
func NewSendRequest(r io.Reader) (req SendRequest, signer string, err error) {
|
|
body, err := ioutil.ReadAll(r)
|
|
if err != nil {
|
|
return
|
|
}
|
|
out, key, err := salty.Verify(body)
|
|
if err != nil {
|
|
return
|
|
}
|
|
signer = key.ID().String()
|
|
err = json.Unmarshal(out, &req)
|
|
return
|
|
}
|
|
|
|
// AvatarRequest is the request used by clients to send messages via a broker
|
|
type AvatarRequest struct {
|
|
Addr *Addr
|
|
Content []byte
|
|
}
|
|
|
|
// NewAvatarRequest reads the signed request body from a client, verifies its signature
|
|
// and returns the resulting `AvatarRequest` and key used to sign the request on success
|
|
// otherwise an empty object and en error on failure.
|
|
func NewAvatarRequest(r io.Reader) (req AvatarRequest, signer string, err error) {
|
|
body, err := ioutil.ReadAll(r)
|
|
if err != nil {
|
|
return
|
|
}
|
|
out, key, err := salty.Verify(body)
|
|
if err != nil {
|
|
return
|
|
}
|
|
signer = key.ID().String()
|
|
err = json.Unmarshal(out, &req)
|
|
return
|
|
}
|