mirror of
https://git.mills.io/saltyim/saltyim.git
synced 2024-07-03 00:33:38 +00:00
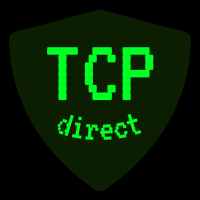
Co-authored-by: mlctrez <mlctrez@gmail.com> Reviewed-on: https://git.mills.io/saltyim/saltyim/pulls/157 Reviewed-by: James Mills <james@mills.io> Co-authored-by: mlctrez <mlctrez@noreply@mills.io> Co-committed-by: mlctrez <mlctrez@noreply@mills.io>
82 lines
2.0 KiB
Go
82 lines
2.0 KiB
Go
package components
|
|
|
|
import (
|
|
"github.com/maxence-charriere/go-app/v9/pkg/app"
|
|
"github.com/mlctrez/goapp-mdc/pkg/drawer"
|
|
"github.com/mlctrez/goapp-mdc/pkg/icon"
|
|
"github.com/mlctrez/goapp-mdc/pkg/list"
|
|
"go.mills.io/saltyim/internal/pwa/storage"
|
|
)
|
|
|
|
const (
|
|
navigationUpdateAction = "navigation.update.action"
|
|
)
|
|
|
|
var DrawerOpen bool
|
|
|
|
type Navigation struct {
|
|
app.Compo
|
|
Type drawer.Type
|
|
drawer *drawer.Drawer
|
|
items list.Items
|
|
Contacts []string
|
|
}
|
|
|
|
func (n *Navigation) HasContact(addr string) bool {
|
|
for _, contact := range n.Contacts {
|
|
if contact == addr {
|
|
return true
|
|
}
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (n *Navigation) Render() app.UI {
|
|
if n.drawer == nil {
|
|
n.drawer = &drawer.Drawer{
|
|
Type: n.Type,
|
|
Id: "navigationDrawer",
|
|
List: &list.List{Type: list.Navigation, Id: "navigationList"},
|
|
}
|
|
}
|
|
items := list.Items{
|
|
{Type: list.ItemTypeAnchor, Graphic: icon.MISettings, Href: "/config", Text: "Settings"},
|
|
{Type: list.ItemTypeAnchor, Graphic: icon.MIPersonAdd, Href: "/newchat", Text: "New Chat"},
|
|
{Type: list.ItemTypeDivider},
|
|
}
|
|
for _, contact := range n.Contacts {
|
|
i := &list.Item{Type: list.ItemTypeAnchor, Graphic: icon.MIPerson, Href: "/#" + contact, Text: contact}
|
|
items = append(items, i)
|
|
}
|
|
n.drawer.List.Items = items.UIList()
|
|
|
|
return n.drawer
|
|
}
|
|
|
|
func (n *Navigation) LoadFromStorage(ctx app.Context) {
|
|
n.Contacts = storage.ContactsLocalStorage(ctx).List()
|
|
}
|
|
|
|
func (n *Navigation) OnMount(ctx app.Context) {
|
|
n.items.SelectHref(ctx.Page().URL().Path)
|
|
ctx.Handle(string(list.Select), func(context app.Context, action app.Action) {
|
|
if action.Value == n.drawer.List {
|
|
if !DesktopMode {
|
|
n.drawer.ActionClose(context)
|
|
DrawerOpen = false
|
|
}
|
|
}
|
|
})
|
|
ctx.Handle(navigationUpdateAction, n.navigationUpdate)
|
|
ctx.Defer(func(context app.Context) { NavigationUpdate(context) })
|
|
}
|
|
|
|
func (n *Navigation) navigationUpdate(ctx app.Context, action app.Action) {
|
|
n.LoadFromStorage(ctx)
|
|
n.drawer.List.Update()
|
|
}
|
|
|
|
func NavigationUpdate(ctx app.Context) {
|
|
ctx.NewAction(navigationUpdateAction)
|
|
}
|