mirror of
https://git.mills.io/saltyim/saltyim.git
synced 2024-06-30 18:51:03 +00:00
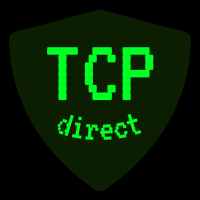
Co-authored-by: James Mills <prologic@shortcircuit.net.au> Reviewed-on: https://git.mills.io/prologic/saltyim/pulls/38
61 lines
1.2 KiB
Go
61 lines
1.2 KiB
Go
package main
|
|
|
|
import (
|
|
"encoding/json"
|
|
"fmt"
|
|
"os"
|
|
"strings"
|
|
|
|
"github.com/spf13/cobra"
|
|
|
|
"go.mills.io/saltyim"
|
|
)
|
|
|
|
var lookupCmd = &cobra.Command{
|
|
Use: "lookup <user>",
|
|
Short: "Lookup a user's config",
|
|
Long: `This command attempts to lookup the user's Salty Config by using
|
|
the Salty IM Discovery process by making a request to the user's Well-Known URI
|
|
|
|
The User is expected to have a Configuration file located at a Well-Known URI of:
|
|
/.well-known/salty/<sha256hex(user@domain.tld)>.json
|
|
- or -
|
|
/.well-known/salty/<user>.json
|
|
|
|
|
|
For example:
|
|
|
|
https://mills.io/.well-known/salty/prologic.json`,
|
|
Args: cobra.MinimumNArgs(1),
|
|
Run: func(cmd *cobra.Command, args []string) {
|
|
user := args[0]
|
|
lookup(user)
|
|
},
|
|
}
|
|
|
|
func init() {
|
|
rootCmd.AddCommand(lookupCmd)
|
|
}
|
|
|
|
func lookup(user string) {
|
|
user = strings.TrimSpace(user)
|
|
if user == "" {
|
|
fmt.Fprintln(os.Stderr, "error no user supplied")
|
|
os.Exit(2)
|
|
}
|
|
|
|
config, err := saltyim.Lookup(user)
|
|
if err != nil {
|
|
fmt.Fprintf(os.Stderr, "error looking up user %s: %s\n", user, err)
|
|
os.Exit(2)
|
|
}
|
|
|
|
data, err := json.Marshal(config)
|
|
if err != nil {
|
|
fmt.Fprintf(os.Stderr, "error parsing lookup response: %s\n", err)
|
|
os.Exit(2)
|
|
}
|
|
|
|
fmt.Fprintln(os.Stdout, string(data))
|
|
}
|