mirror of
https://git.mills.io/saltyim/saltyim.git
synced 2024-06-30 18:51:03 +00:00
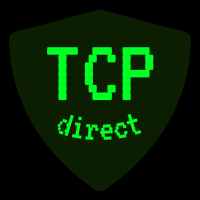
Co-authored-by: James Mills <prologic@shortcircuit.net.au> Reviewed-on: https://git.mills.io/prologic/saltyim/pulls/46
94 lines
2.0 KiB
Go
94 lines
2.0 KiB
Go
package main
|
|
|
|
import (
|
|
"fmt"
|
|
"io"
|
|
"io/ioutil"
|
|
"os"
|
|
"strings"
|
|
|
|
"github.com/spf13/cobra"
|
|
"github.com/spf13/viper"
|
|
|
|
"go.mills.io/saltyim"
|
|
)
|
|
|
|
const (
|
|
maxMessageSize = 1 << 12 // 4kB
|
|
)
|
|
|
|
var sendCmd = &cobra.Command{
|
|
Use: "send <user> [<message>]",
|
|
Short: "Send a message to a user",
|
|
Long: `This command attempts to lookup the user's Salty Config by using
|
|
the Salty IM Discovery process by making a request to the user's Well-Known URI
|
|
After it will attempt to send the message via a HTTP POST to their Endpoint.
|
|
|
|
The User is expected to have a Configuration file located at a Well-Known URI of:
|
|
/.well-known/salty/<sha256hex(user@domain.tld)>.json
|
|
- or -
|
|
/.well-known/salty/<user>.json
|
|
|
|
For example:
|
|
|
|
https://mills.io/.well-known/salty/prologic.json`,
|
|
Args: cobra.MinimumNArgs(1),
|
|
Run: func(cmd *cobra.Command, args []string) {
|
|
user := viper.GetString("user")
|
|
identity := viper.GetString("identity")
|
|
|
|
var profiles []profile
|
|
viper.UnmarshalKey("profiles", &profiles)
|
|
for _, p := range profiles {
|
|
if user == p.User {
|
|
identity = p.Identity
|
|
}
|
|
}
|
|
|
|
me := &saltyim.Addr{}
|
|
if sp := strings.Split(user, "@"); len(sp) > 1 {
|
|
me.User = sp[0]
|
|
me.Domain = sp[1]
|
|
}
|
|
// XXX: What if me.IsZero()
|
|
|
|
send(me, identity, args[0], args[1:]...)
|
|
},
|
|
}
|
|
|
|
func init() {
|
|
rootCmd.AddCommand(sendCmd)
|
|
}
|
|
|
|
func send(me *saltyim.Addr, identity, user string, args ...string) {
|
|
user = strings.TrimSpace(user)
|
|
if user == "" {
|
|
fmt.Fprintf(os.Stderr, "error: no user supplied\n")
|
|
os.Exit(2)
|
|
}
|
|
|
|
cli, err := saltyim.NewClient(me, identity)
|
|
if err != nil {
|
|
fmt.Fprintf(os.Stderr, "error initializing client: %s\n", err)
|
|
os.Exit(2)
|
|
}
|
|
|
|
var msg string
|
|
|
|
if len(args) == 0 {
|
|
data, err := ioutil.ReadAll(io.LimitReader(os.Stdin, maxMessageSize))
|
|
if err != nil {
|
|
fmt.Fprintf(os.Stderr, "error reading message from stdin: %s\n", err)
|
|
os.Exit(2)
|
|
}
|
|
msg = string(data)
|
|
} else {
|
|
msg = strings.Join(args, " ")
|
|
}
|
|
|
|
if err := cli.Send(user, msg); err != nil {
|
|
fmt.Fprintf(os.Stderr, "error sending message to %s: %s\n", user, err)
|
|
os.Exit(2)
|
|
}
|
|
}
|