mirror of
https://git.mills.io/saltyim/saltyim.git
synced 2024-06-28 09:41:02 +00:00
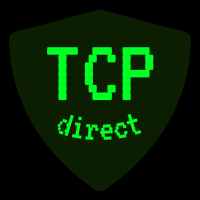
feat: add compression negotiation for sent messages fix: unix homedir handling the service will negotiate a compression algo for sending messages when a user chats someone during the auto discovery, the service returns an `Accept-Encoding: br, gzip, deflate` the client saves that response and so when it makes POSTs of messages adds the best `Content-Encoding` and compresses the message example: ``` >> GET /.well-known/salty/c765c69040d98f3af2181237f47ec01398d80f8ab2690fe929e4311ab05dec01.json << Accept-Encoding: br, gzip, deflate << << {"endpoint":"https://salty.home.arpa/inbox/01FZBR8Y2E6TH949JA3925WF71","key":"kex1wurry09ftqjuxgjl0jxmqypv4axqvzqljkgeadxjcpwtfuhcedcslck52d"} >> POST /inbox/01FZBR8Y2E6TH949JA3925WF71 >> Content-Encoding: br >> >> [Brotli Compressed data] ``` this PR depends on https://git.mills.io/prologic/msgbus/pulls/24 Co-authored-by: Jon Lundy <jon@xuu.cc> Reviewed-on: https://git.mills.io/saltyim/saltyim/pulls/91 Co-authored-by: xuu <xuu@noreply@mills.io> Co-committed-by: xuu <xuu@noreply@mills.io>
53 lines
1003 B
Go
53 lines
1003 B
Go
package internal
|
|
|
|
import (
|
|
"encoding/json"
|
|
"fmt"
|
|
"os"
|
|
"path/filepath"
|
|
|
|
"go.mills.io/saltyim"
|
|
)
|
|
|
|
const (
|
|
wellknownPath = ".well-known/salty"
|
|
)
|
|
|
|
func CreateConfig(conf *Config, hash string, key string) error {
|
|
p := filepath.Join(conf.Data, wellknownPath)
|
|
p = saltyim.FixUnixHome(p)
|
|
|
|
fn := filepath.Join(p, fmt.Sprintf("%s.json", hash))
|
|
if FileExists(fn) {
|
|
return nil
|
|
}
|
|
|
|
if err := os.MkdirAll(p, 0755); err != nil {
|
|
return fmt.Errorf("error creating config paths %s: %w", p, err)
|
|
}
|
|
|
|
ulid, err := saltyim.GenerateULID()
|
|
if err != nil {
|
|
return fmt.Errorf("error generating ulid")
|
|
}
|
|
|
|
endpointURL := *conf.baseURL
|
|
endpointURL.Path = fmt.Sprintf("/inbox/%s", ulid)
|
|
|
|
config := saltyim.Config{
|
|
Endpoint: endpointURL.String(),
|
|
Key: key,
|
|
}
|
|
|
|
data, err := json.Marshal(config)
|
|
if err != nil {
|
|
return fmt.Errorf("error serializing config")
|
|
}
|
|
|
|
if err := os.WriteFile(fn, data, 0644); err != nil {
|
|
return fmt.Errorf("error writing config to %s: %w", fn, err)
|
|
}
|
|
|
|
return nil
|
|
}
|