mirror of
https://github.com/biergaizi/codecrypt
synced 2024-06-16 03:48:19 +00:00
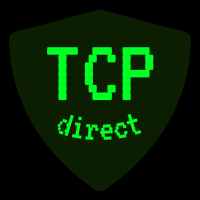
Previously, we assume the existence of a incomplete block at end of the input. However, it's possible that input's an exact multiple of block size. In this case, the first argument of process_final_incomplete_block() will be one-past-the-last element, the second argument will be zero. This' an ill-defined call, and it will trigger an assertion failure of std::vector Assertion '__builtin_expect(__n < this->size(), true)' failed. This commit introduced a check. If we see the length of the last incomplete block is zero, we call process_final_incomplete_block(NULL, 0); which immediately finalizes CubeHash without hashing additional data. Signed-off-by: Tom Li <tomli@tomli.me>
111 lines
2.8 KiB
C++
111 lines
2.8 KiB
C++
|
|
/*
|
|
* This file is part of Codecrypt.
|
|
*
|
|
* Copyright (C) 2013-2016 Mirek Kratochvil <exa.exa@gmail.com>
|
|
*
|
|
* Codecrypt is free software: you can redistribute it and/or modify it
|
|
* under the terms of the GNU Lesser General Public License as published by
|
|
* the Free Software Foundation, either version 3 of the License, or (at
|
|
* your option) any later version.
|
|
*
|
|
* Codecrypt is distributed in the hope that it will be useful, but WITHOUT
|
|
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
|
|
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public
|
|
* License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public License
|
|
* along with Codecrypt. If not, see <http://www.gnu.org/licenses/>.
|
|
*/
|
|
|
|
#include "cubehash_impl.h"
|
|
|
|
#include "hash.h"
|
|
|
|
template<int I, int R, int B, int F, int H>
|
|
class cubehash : public hash_func
|
|
{
|
|
public:
|
|
uint size() {
|
|
return H;
|
|
}
|
|
|
|
std::vector<byte> operator() (const std::vector<byte>&a) {
|
|
cubehash_state<I, R, B, F, H> state;
|
|
size_t i;
|
|
|
|
state.init();
|
|
|
|
for (i = 0; i + B <= a.size(); i += B)
|
|
state.process_block (& (a[i]));
|
|
|
|
if (a.size() - i != 0)
|
|
state.process_final_incomplete_block (& (a[i]), a.size() - i);
|
|
else
|
|
state.process_final_incomplete_block (NULL, 0); //empty block, just finalize
|
|
|
|
std::vector<byte> result;
|
|
result.resize (H, 0);
|
|
state.get_hash (& (result[0]));
|
|
return result;
|
|
}
|
|
};
|
|
|
|
template<int I, int R, int B, int F, int H>
|
|
class cubehashproc : public hash_proc
|
|
{
|
|
cubehash_state<I, R, B, F, H> state;
|
|
byte buf[B];
|
|
int bpos;
|
|
public:
|
|
uint size() {
|
|
return H;
|
|
}
|
|
|
|
void init() {
|
|
state.init();
|
|
bpos = 0;
|
|
}
|
|
|
|
void eat (const byte*a, const byte*aend) {
|
|
int apos = 0;
|
|
int asize = aend - a;
|
|
if (bpos) {
|
|
for (; bpos < B && apos < asize; ++bpos, ++apos)
|
|
buf[bpos] = a[apos];
|
|
if (bpos == B) {
|
|
state.process_block (buf);
|
|
bpos = 0;
|
|
}
|
|
}
|
|
while (apos + B <= asize) {
|
|
state.process_block (& (a[apos]));
|
|
apos += B;
|
|
}
|
|
for (; apos < asize; ++apos, ++bpos)
|
|
buf[bpos] = a[apos];
|
|
}
|
|
|
|
std::vector<byte> finish() {
|
|
state.process_final_incomplete_block (buf, bpos);
|
|
std::vector<byte> result;
|
|
result.resize (H, 0);
|
|
state.get_hash (& (result[0]));
|
|
return result;
|
|
}
|
|
};
|
|
|
|
|
|
|
|
class cube128hash : public cubehash<16, 16, 32, 32, 16> {};
|
|
class cube192hash : public cubehash<16, 16, 32, 32, 24> {};
|
|
class cube256hash : public cubehash<16, 16, 32, 32, 32> {};
|
|
class cube384hash : public cubehash<16, 16, 32, 32, 48> {};
|
|
class cube512hash : public cubehash<16, 16, 32, 32, 64> {};
|
|
|
|
class cube128proc : public cubehashproc<16, 16, 32, 32, 16> {};
|
|
class cube192proc : public cubehashproc<16, 16, 32, 32, 24> {};
|
|
class cube256proc : public cubehashproc<16, 16, 32, 32, 32> {};
|
|
class cube384proc : public cubehashproc<16, 16, 32, 32, 48> {};
|
|
class cube512proc : public cubehashproc<16, 16, 32, 32, 64> {};
|