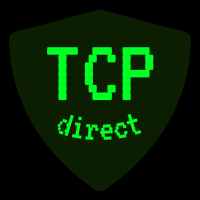
0. Maximum parity with upstream code 1. Added Apache-required modification notices 2. Added Apache license
61 lines
1.0 KiB
Go
61 lines
1.0 KiB
Go
// Copyright 2014-2018 Grafana Labs
|
|
// Released under the Apache 2.0 license
|
|
|
|
package ldap
|
|
|
|
import (
|
|
"strings"
|
|
|
|
ldap "github.com/go-ldap/ldap/v3"
|
|
)
|
|
|
|
func isMemberOf(memberOf []string, group string) bool {
|
|
if group == "*" {
|
|
return true
|
|
}
|
|
|
|
for _, member := range memberOf {
|
|
if strings.EqualFold(member, group) {
|
|
return true
|
|
}
|
|
}
|
|
return false
|
|
}
|
|
|
|
func getArrayAttribute(name string, entry *ldap.Entry) []string {
|
|
if strings.ToLower(name) == "dn" {
|
|
return []string{entry.DN}
|
|
}
|
|
|
|
for _, attr := range entry.Attributes {
|
|
if attr.Name == name && len(attr.Values) > 0 {
|
|
return attr.Values
|
|
}
|
|
}
|
|
return []string{}
|
|
}
|
|
|
|
func getAttribute(name string, entry *ldap.Entry) string {
|
|
if strings.ToLower(name) == "dn" {
|
|
return entry.DN
|
|
}
|
|
|
|
for _, attr := range entry.Attributes {
|
|
if attr.Name == name {
|
|
if len(attr.Values) > 0 {
|
|
return attr.Values[0]
|
|
}
|
|
}
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func appendIfNotEmpty(slice []string, values ...string) []string {
|
|
for _, v := range values {
|
|
if v != "" {
|
|
slice = append(slice, v)
|
|
}
|
|
}
|
|
return slice
|
|
}
|