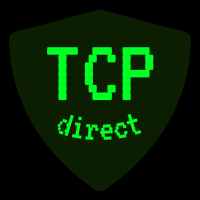
* telnet module should not return success when it finds something other than telnet * telnet module should not return success when it finds something other than telnet * Adds verification step for POP3 banners * Add validation for IMAP banners & fix some formatting problems * Verify SMTP banners exist and are successful * Add check for is_dnp3 flag which seems to be working as expected * Fix dropping SCAN_APPLICATION_ERROR in IMAP * Fix dropping SCAN_APPLICATION_ERROR in POP3 * Fix dropping SCAN_APPLICATION_ERROR in SMTP * Add protocol and blacklist indicators to email protocols Co-authored-by: Elliot Cubit <elliotcubit@elliots-mbp.lan>
39 lines
1005 B
Go
39 lines
1005 B
Go
package imap
|
|
|
|
import (
|
|
"net"
|
|
"regexp"
|
|
"io"
|
|
|
|
"github.com/zmap/zgrab2"
|
|
)
|
|
|
|
// This is the regex used in zgrab.
|
|
var imapStatusEndRegex = regexp.MustCompile(`\r\n$`)
|
|
|
|
const readBufferSize int = 0x10000
|
|
|
|
// Connection wraps the state and access to the SMTP connection.
|
|
type Connection struct {
|
|
Conn net.Conn
|
|
}
|
|
|
|
// ReadResponse reads from the connection until it matches the imapEndRegex. Copied from the original zgrab.
|
|
// TODO: Catch corner cases
|
|
func (conn *Connection) ReadResponse() (string, error) {
|
|
ret := make([]byte, readBufferSize)
|
|
n, err := zgrab2.ReadUntilRegex(conn.Conn, ret, imapStatusEndRegex)
|
|
if err != nil && err != io.EOF && !zgrab2.IsTimeoutError(err) {
|
|
return "", err
|
|
}
|
|
return string(ret[:n]), nil
|
|
}
|
|
|
|
// SendCommand sends a command, followed by a CRLF, then wait for / read the server's response.
|
|
func (conn *Connection) SendCommand(cmd string) (string, error) {
|
|
if _, err := conn.Conn.Write([]byte(cmd + "\r\n")); err != nil {
|
|
return "", err
|
|
}
|
|
return conn.ReadResponse()
|
|
}
|