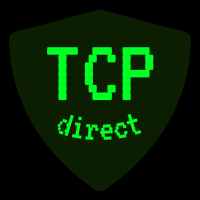
* Changes grab to return *ScanResults. Implements ippInContentType correctly. * Slots in an operational re-working of several HTTP module functions, and adds dependency on zgrab's http module. Includes some laregly copy-pasted sections worthy of scrutiny. * Adds support to retry failed HTTP over HTTPS. Removes vestigial functions. * Implements sending CUPS-get-printers request if CUPS is detected, yielding more detailed & accurate version information. Also handles URI's more correctly. * Creates separate container to run IPP over TLS on CUPS. Runs basic tests against both containers. * Creates virtual printer on each container to test for augmenting data with CUPS-get-printers request (which only works when printers exist). * Augments version information with CUPS-get-printers response if possible. * Allows specifying IPP version in constructed requests. Checks for version-not-supported server error. * Allows resending IPP requests with different versions if we hit a version-not-supported error. * Updates IPP zgrab2 schema to include fields added in modules/ipp/scanner.go * Removes unnecessary TODO's * Updates testable example for new definition of AttributeByteString * Removes versionNotSupported's dependency on bufferFromBody. Checks bounds on generated requests' fields correctly. * Updates zgrab2 IPP schema to match ScanResults object in modules/ipp/scanner.go * Corrects IPP tests, bounds checking, zgrab schema formatting. * Logs errors for unexpected behavior in buffer io operations. Updates schema to include standalone fields for attributes described in CUPS-get-printers response. * Logs at debug level only when verbose flag is set. Prints accurate error message when CUPSVersion test fails. * Handles HTTP request errors before checking for nil response/body. Fixes and tests convertURIToIPP.
33 lines
1.2 KiB
Go
33 lines
1.2 KiB
Go
package ipp
|
|
|
|
import (
|
|
"bytes"
|
|
"fmt"
|
|
)
|
|
|
|
func ExampleAttributeByteString() {
|
|
var buf bytes.Buffer
|
|
if err := AttributeByteString(0x47, "attributes-charset", "us-ascii", &buf); err == nil {
|
|
fmt.Println(buf.Bytes())
|
|
}
|
|
// Output: [71 0 18 97 116 116 114 105 98 117 116 101 115 45 99 104 97 114 115 101 116 0 8 117 115 45 97 115 99 105 105]
|
|
}
|
|
|
|
func ExampleConvertURIToIPP() {
|
|
fmt.Println(ConvertURIToIPP("http://www.google.com:631/ipp", false))
|
|
fmt.Println(ConvertURIToIPP("https://www.google.com:631/ipp", true))
|
|
fmt.Println(ConvertURIToIPP("http://www.google.com/ipp", false))
|
|
fmt.Println(ConvertURIToIPP("https://www.google.com/ipp", true))
|
|
fmt.Println(ConvertURIToIPP("http://www.google.com:631", false))
|
|
fmt.Println(ConvertURIToIPP("https://www.google.com:631", true))
|
|
// TODO: Eventually test for scheme-less urls, but getHTTPURL will never construct one
|
|
//fmt.Println(ConvertURIToIPP("www.google.com:631/ipp", false))
|
|
//fmt.Println(ConvertURIToIPP("www.google.com:631/ipp", true))
|
|
// Output:
|
|
// ipp://www.google.com:631/ipp
|
|
// ipps://www.google.com:631/ipp
|
|
// ipp://www.google.com:631/ipp
|
|
// ipps://www.google.com:631/ipp
|
|
// ipp://www.google.com:631
|
|
// ipps://www.google.com:631
|
|
} |