mirror of
https://github.com/cberner/raptorq.git
synced 2024-06-16 11:59:01 +00:00
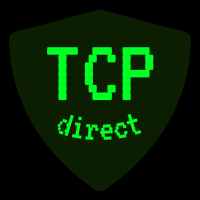
* Add CPython wrapper * Add CPython wrapper to build pipeline * Don't use Travis CI matrix expansion * Install maturin and add cache * Install Python * Install fix incorrect package name 'pip3' * Update package list * Split build in two jobs * Don't cache as installing lichking will error otherwise * Don't lint on nightly * Add Python tests * Add venv * Fix execution order * Run linter * Run linter
39 lines
1.1 KiB
Python
39 lines
1.1 KiB
Python
import os
|
|
import random
|
|
from raptorq import Encoder, Decoder
|
|
|
|
|
|
def main():
|
|
# Generate some random data to send
|
|
data = os.urandom(10000)
|
|
|
|
# Create the Encoder, with an MTU of 1400 (common for Ethernet)
|
|
encoder = Encoder.with_defaults(data, 1400)
|
|
|
|
# Perform the encoding, and serialize to bytes for transmission
|
|
packets = encoder.get_encoded_packets(15)
|
|
|
|
# Here we simulate losing 10 of the packets randomly. Normally, you would send them over
|
|
# (potentially lossy) network here.
|
|
random.shuffle(packets)
|
|
# Erase 10 packets at random
|
|
packets = packets[:-10]
|
|
|
|
# The Decoder MUST be constructed with the configuration of the Encoder.
|
|
# The configuration should be transmitted over a reliable channel
|
|
decoder = Decoder.with_defaults(len(data), 1400)
|
|
|
|
# Perform the decoding
|
|
result = None
|
|
for packet in packets:
|
|
result = decoder.decode(packet)
|
|
if result is not None:
|
|
break
|
|
|
|
# Check that even though some of the data was lost we are able to reconstruct the original message
|
|
assert result == data
|
|
|
|
|
|
if __name__ == '__main__':
|
|
main()
|